Initial Setup
Lesson 11 Chapter 3 Module 2
We're now going to turn our attention to building out the frontend of our fungible token project. In your project folder, all the frontend development happens inside the src folder so expand that and let's take a look at each of the files present in the src root.
index.html - Setting the Project Title and Description
Open up the index.html file. This is the entry point of the app. We don't need to do too much here - simply change the app title and add a description.
Look for <title>Welcome to Near with React<title>. That sets the title of your app and is what gets displayed in a browser tab. Change it to whatever you want to call your project - I'm going to name mine Vital Point Coin.
Also in the <head> of index.html, we're going to add another <meta> tag with a description of the project. When done, you're <head></head> section should look something like this:
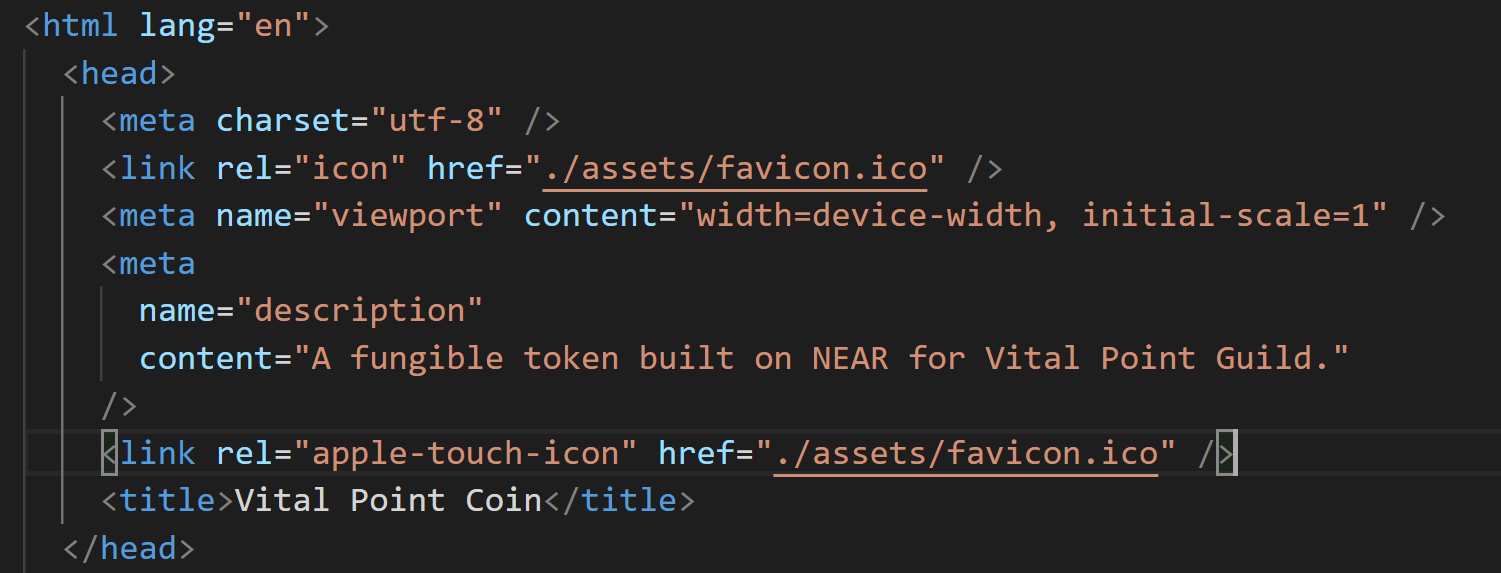
The only other thing to take note of in the index.html file is the <div id='root'></div> tag. React uses this tag to know where to start rendering the app components we're going to build. And with that, we're done with index.html.
index.js
Index.js is the entry point to our Dapp. It calls the contract initialization function and basically sets things up before serving up the App itself.
import React from 'react'
import ReactDOM from 'react-dom'
import App from './App'
import { initContract } from './utils'
window.nearInitPromise = initContract()
.then(() => {
ReactDOM.render(
<App />,
document.querySelector(‘#root’)
)
})
.catch(console.error)
This is a pretty short file, so open it up for a quick run through. At the top, we're importing React and ReactDom which are the React libraries we're using to build things out.
Next we import App which is a React functional component that gets rendered further below in the ReactDom.render section. You might remember #root from index.html? That's the div id and this basically says look for that tag and then render (display) the App component where you find it.
We're also importing initContract from utils.js in the same directory. Thats an asynchronous initiatlization function that we'll take a look at shortly - it's used to create the connection to the NEAR blockchain.
The codeblock starting with window.nearInitPromise starts up the app by calling the initContract() function. Because it's asynchronous, it runs and returns a promise. When the promise is received/resolved (indicating that initialization is done) we move on to the rendering actions. If there is any error, it is caught by the .catch(console.error) statement and displayed to the console. (open developer tools in your browser to see).
Before we move on, we're going to add some routing ability to the app so that we can change pathnames. We do that by installing react-router-dom. In your terminal type:
npm install react-router-dom --save
Then we'll change index.js to import BrowserRouter and Route from that package and wire it up. Change the file to look like the following:
import React from 'react'
import ReactDOM from 'react-dom'
import { BrowserRouter, Route } from "react-router-dom";
import App from './App'
import { initContract } from './utils'
window.nearInitPromise = initContract()
.then(() => {
ReactDOM.render(
<BrowserRouter>
<Route path=”/” component={App} />
</BrowserRouter>,
document.querySelector(‘#root’)
)
})
.catch(console.error)
Wrapping our routes in the BrowserRouter component gives us access to route, location, pathname, and history information in the Dapp. The Route path part of this in plain English says that when we visit the root url (e.g. localhost/) - return the App component. Similarly, we can define additional routes and serve up specific components at each one.
config.js - Network Configurations
We need to specify some configuration information to connect our app to the contract that we've deployed on NEAR. Depending on whether we are in production or development will dictate which network configuration in config.js is used to make that connection.
When we were setting up our project way back at the start we created a .env file and added it to .gitignore (so our secrets don't end up published to the world). In that file we need to define three environment variables. The env-cmd package that is a package installed as part of the overall project looks in .env at the start and sets up the environment to be able to use these variables. They are accessible via process.env.variablename. The three environment variables we want to define are:
- CONTRACT_NAME = vpc.vitalpointai.testnet (note: this value is the name of the contract account you deployed your fungible token contract to)
- NODE_ENV = development (note: the value here can be development, production, or test. If it is not set, development is the default. Because we deployed our contract to testnet, we want to work in the development environment which connects to testnet by default)
- DEFAULT_GAS_VALUE = 300000000000000 (note: when we send transactions to the blockchain, there is a cost associated with any state changes - they are paid with this default gas value and any remainder is returned back to your account - this is a very small amount (believe it's 30 TGas) considering 1 near has 10^24 yocto - it's significantly smaller than any transaction cost you'd get on something like Ethereum or Bitcoin).
If you look back in the config.js file under case 'development': - you'll see what is returned when getConfig(env) is called (where env = development). While you're not too concerned with these values - it's good to know where things like the networkId are defined. walletUrl is the home of the NEAR wallet used to create accounts and so on. Most importantly is the nodeUrl which is the gateway to NEAR and your contract that will be used.
utils.js - Initializing the Contract Backend
utils.js does the heavy lifting of initializing things for your app to connect to your contract and use the functions in it. The comments are pretty good at describing what each particular section does and you won't need to change most of this unless you're setting up some kind of custom configuration.
Notice the imports from near-api-js. You'll rely heavily on that API as you build applications that interact with the NEAR blockchain.
What you will need to change are the change and view methods listed under the window.contract initialization. Specifically, you need to list all the view and change methods that we created in our backend main.ts contract in their requisite sections. To that end:
1. replace viewMethods: ['getGreeting'], with your view methods from your main.ts contract in assembly directory:
viewMethods: [
'isOwner',
'get_total_supply',
'get_balance',
'get_allowance',
'getTokenName',
'getSymbolName',
'getPrecision',
'getInitialSupply',
'getOwner',
'getInit'
],
2. replace changeMethods: ['setGreeting'], with your change methods from your main.ts contract in assembly directory:
changeMethods: [
'init',
'inc_allowance',
'dec_allowance',
'transfer_from',
'transfer',
'burn',
'mint'
'transferOwnership'
],
Next, there is currently a function (onSubmit(event)) that is in the utils.js as part of the example app that was deployed. We'll delete it.
Following that, you'll see a export function logout() and export function login(). Those are used to log you in/out of your NEAR account to interact with the app. Leave them as be.
Rest of the files/directories in ./src
- global.css - contains global style information - can leave as is for now
- jest.init.js - used as part of testing - leave as is
- main.test.js - where tests will eventually go - we'll cover them later
- /assets folder - typically where you'll store images or other media that your application uses
- wallet/login (and contents) folders - go ahead and delete them - you won't need them.
There is still one file we haven't talked about - App.js. That's going to be a bit more in depth, so we'll pick it up in the next lesson.